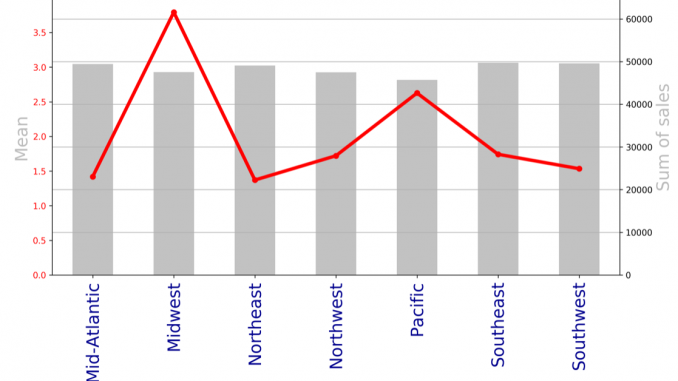
In [1]:
import pandas as pd
import matplotlib.pyplot as plt
import numpy as np
import seaborn as sns
df = pd.read_csv('c:/1/WA_Fn-UseC_-Sales-Win-Loss.csv')
df.head()
Out[1]:
In [2]:
SKS = df.pivot_table(index='Region',values='Sales Stage Change Count', aggfunc=['sum','mean']).reset_index()
SKS
Out[2]:
In [3]:
SKS.columns
Out[3]:
Merge column names
In [4]:
SKS.columns = ['_'.join(col) for col in SKS.columns.values]
SKS
Out[4]:
Combiplot
In [10]:
fig = plt.figure(figsize=(12,6), dpi= 280)
ax = SKS['mean_Sales Stage Change Count'].plot(kind="bar", color='darkgrey',alpha=0.7)
ax.set_ylim(0,1.3*SKS["mean_Sales Stage Change Count"].max())
ax.set_xlabel("Regions", color='darkgrey',alpha=0.8, fontsize=20)
ax.set_ylabel(r"Mean", color='darkgrey',alpha=0.8, fontsize=20)
ax.grid(False)
ax.tick_params(axis='y', rotation=0, labelcolor='red')
ax.tick_params(axis='x', rotation=90, labelcolor='darkblue')
ax.set_xticklabels(SKS['Region_'], rotation=90, fontdict={'fontsize':20})
ax2 = ax.twinx()
ax2.plot(ax.get_xticks(),SKS['sum_Sales Stage Change Count'],'--', marker='o', c='red', linewidth=6)
ax2.set_ylim(0,1.05*SKS["sum_Sales Stage Change Count"].max())
ax2.grid(True)
ax2.set_ylabel(r"Sum of sales", color='darkgrey',alpha=0.8, fontsize=20)
ax2.set_title("Sales by regions", fontsize=23, alpha=0.4)
plt.show()